Why Kotlin?
Kotlin is the new kid on the block in terms of JVM based languages, but what is making this newcomer convert Java developers where others have not?
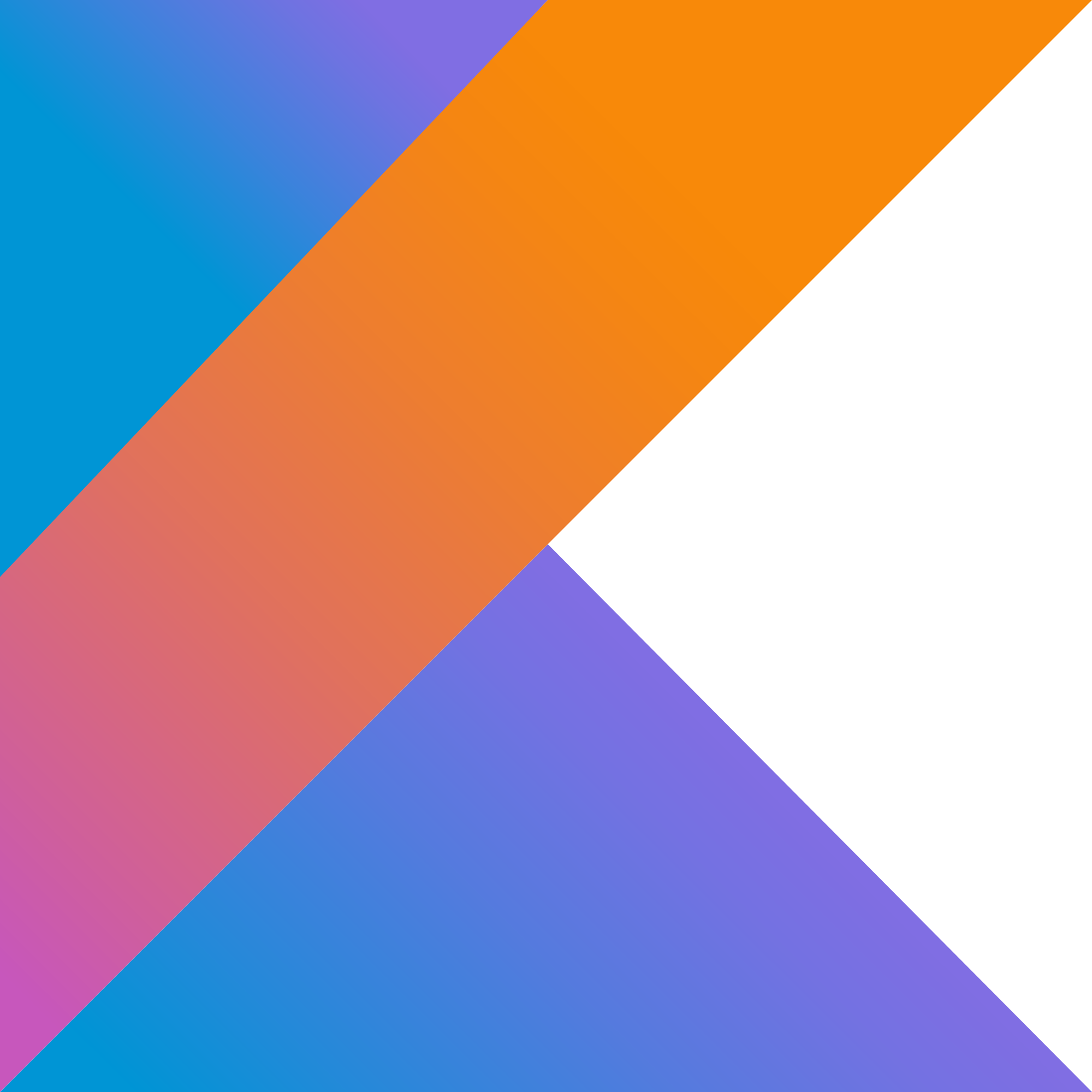
Kotlin was created by the makers of IntelliJ, JetBrains. The name is derived from the city in Russia in which most of the developers were based. It is becoming popular and taking over as Java developers turn to it in droves. Why should we pay attention to this new language?
Kotlin has gained a lot of popularity since its release in 2016 and is suitable for both Android and server side development. It runs on the JVM and interoperability with Java has always been a design goal. I wanted to take a look at the language to see why it has become so popular.
I’ve spoken to Java developers who profess love for their main language, but who prefer Kotlin. The language focuses on being more concise than Java, but still keeping type strictness. This is unlike some of the of the JVM family of languages that have sprung up recently, such as Groovy and Scala.
Kotlin is designed so that it can be phased into your existing applications. As you write new code, you can do so in Kotlin and place it alongside older Java code. Everything should work smoothly – it all gets compiled to bytecode after all. And if you are already using IntelliJ for development, the process of switching over should be seamless.
Reasons to Change
So switching to Kotlin should not be a big deal, but before doing so you need to be convinced about its benefits. Here are a few quick points to get started.
Less Code
Kotlin is a lot more concise than Java. Annotation libraries can be added to Java to remove some of the boiler plate code, for example, making POJOs easier to create but this sort of thing is built into Kotlin.
Type inference is built in, allowing you to drop the type definition if the context is clear at compile time. Kotlin is still strongly typed so you will get a compile error if the type is ambiguous.
The Kotlin team estimate that you can save 40% code savings by using their syntax instead of plain Java. That means code that is cleaner and more easily read, should be quicker to write, and will be easier to maintain.
The lack of boiler plate can be demonstrated by comparing the humble Hello World! in both Java and Kotlin.
public class HelloWorld {
public static void main(String args...) {
System.out.println("Hello, World!");
}
}
Kotlin is much more concise. Note that a class is not mandatory:
fun main(args: Array<String>) {
println("Hello, World!")
}
Another simple example uses a POJO with getters and setters. In Kotlin, all that you have to do is to define the properties, there is no need for getters, setters and constructors. This is an example from try.kotlinlang.org which creates a class User
with two properties.
data class User(val name: String, val id: Int)
This definition is enough to build a class with a constructor that takes both values and getter and setter methods for both of the properties. I won’t create the Java version as it is too long and boring to look through. If you want you can write it out for yourself. Note that the data
keyword also produces the following convenient methods: toString()
, equals()
, hashCode()
and copy()
.
Immutability
There are two ways of defining what we would think of as variables in Java, with the keywords val
and var
. The former creates an immutable object and the latter a more traditional variable that can have a new value. Immutable means that the reference cannot be reassigned. It may be possible to modify certain immutable types, such as adding to lists or maps.
This protects against problems during concurrent operations in that allows much simpler code to be written when designing highly parallel applications. You don’t need to worry about different threads modifying a shared object’s value as this is not allowed on immutable objects.
Immutable objects are a key ingredient when building functional programs and while switching from a variable background may be difficult, it is a safer way to program in general. Think of creating new objects rather than changing existing ones. If you are a Java programmer, you have been using immutable objects already, but may not realise.
The Java String
class is a great example of an immutable. Once created it never changes. Every time you append or substring, a new object is created. This may not be obvious in day to day interaction with the code; Kotlin, by being explicit at declaration stage, helps to keep immutability in the front of developers minds. Where possible, use val
type immutable declarations.
String Templates
Strings can be a tricky thing to deal with in Java. Whether you are using the concatenation operator, +
, or using a StringBuilder
, the code is difficult to read, especially when you have to add variables to the contents of the string. The ability to do string replacement exists, with the String.format()
static method, but Kotlin’s approach is cleaner.
Simply add your variables to the string with a $
prefix and your string is built for you.
val dogs = 2
println("I have $dogs dogs")
You can place more complicated expressions by also using chain brackets:
val dogs = 2
val cats = 4
println("I have ${dogs + cats} animals!")
Note also that Kotlin has made println
a top level keyword. This might not be useful within application code where it is unlikely that you will be writing to the console, but it is quick example of how the Kotlin developers have tried to remove boilerplate. Should you really need to type the full System.out.println
every time you want write to the console?
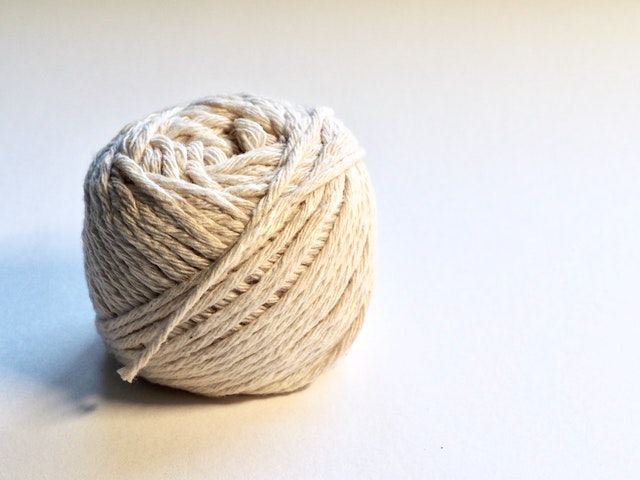
Kotlin also adds a raw string type denoted by triple quotation marks which removes the need to use the string escape character, \
, and allows multi-line strings to be created right inside the class definition. This is especially useful for creating regular expressions and raw SQL.
val multi = """This is a
multi-line string that will can
contain "quatation marks" without
the need or an escape character"""
Conclusion
I found so many reasons to like what the Kotlin creators have created, that I decided to break this post up. We’ve only scratched the surface of what programming goodies are packed inside this concise language.
Update: check out part two here.