Variables in JavaScript
When declaring variables in JavaScript, especially when coming from a Java background, there are a few things to be aware of. Here are a few notes I took.
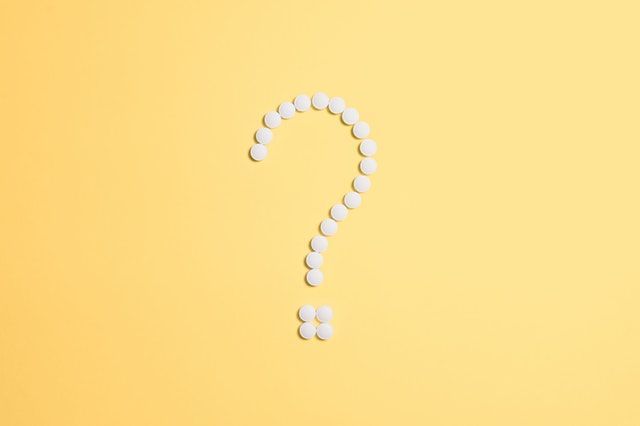
When declaring variables in JavaScript, especially when coming from a Java background, there are a few things to be aware of. Here are a few notes I took.
JavaScript is a recent enough adopter of the const
keyword, its version of a constant, when it was introduced with ES2015. The Let
keyword was also introduced. But first let us look at the standard way to declare a variable.
JavaScript is not strongly typed, and there are several ways to make a declaration. In general it is best to use the var
keyword as follows:
var number = 1;
You don’t have to do so, the following would work also:
number = 1;
However, there is a big difference between the two examples. Take a look at the following example to see if you can spot what happens to the outerScope
variable.
function multiply() {
var innerScope = 10;
outerScope = 35;
console.log( innerScope * outerScope )
}
multiply();
350
innerScope;
Uncaught ReferenceError: innerScope is not defined
at <anonymous>:1:1
(anonymous) @ VM555:1
outerScope;
35
The first variable declared in the function, innerScope
, behaves as we would expect from a Java background; it is only visible within the scope that it has been declared in. However, without the var
keyword, the outerScope
variable behaves differently. It is available to a global scope.
As you can imagine this can lead to unintended consequences if the behaviour is not understood correctly. It can lead to multiple parts of the codebase updating the same, global variables without the others knowing and should be avoided. Always use the var
keyword when declaring variables.
Constants
One thing that irks many non-Java developers is that it can be overly verbose. A pet hate of mine is that constant declarations require two modifiers, static
and final
. And because default visibility is package in Java, we usually add either the public
or private
modifier leading to overly wordy declarations.
JavaScript began life without any constants, but when they were finally introduced, at least the designers had the good sense to do so with a short keyword, const
. Once declared and set, any attempt to update a constant will produce an error:
const c = 10;
c = 11;
VM371:2 Uncaught TypeError: Assignment to constant variable.
at <anonymous>:2:3
(anonymous) @ VM371:2
Let it Be
In Java, tight scoping rules mean that when a variable name is reused, clear rules separate access to the variable. Take for example this method, where the use of the variable within the for loop does not affect the static class variable with the same name.
public class TestScope {
private static int number = 17;
public static void main (String [] args) {
for ( int i = 0; i < 5; i++ ) {
// redeclare and change the number variable
int number = i * 10;
System.out.println("Inner number is: " + number);
}
System.out.println("Outer number is: " + number);
}
}
When run this will produce:
Inner number is: 0
Inner number is: 10
Inner number is: 20
Inner number is: 30
Inner number is: 40
Outer number is: 17
JavaScript behaves a little differently. This simple example illustrates the difference:
var number = 100;
console.log(number);
{
var number = 75;
console.log(number);
}
console.log(number);
100
75
75
The second declaration of the number
variable affects the first and there is no compiler or runtime warning that this is happening. I’m not sure whether this is a feature for some reason, but there is a way around it using the keyword let
should a variable name need to be reused.
var number = 100;
console.log(number);
{
let number = 75;
console.log(number);
}
console.log(number);
100
75
100
Just another thing to be aware of when transitioning from Java to JavaScript. I’ll continue this series of short articles, which can be found under Tech Shorts, as I come across interesting differences.