Spelling Game
In this post I outline a spelling test game that I want to build with my children to introduce them to some programming concepts.
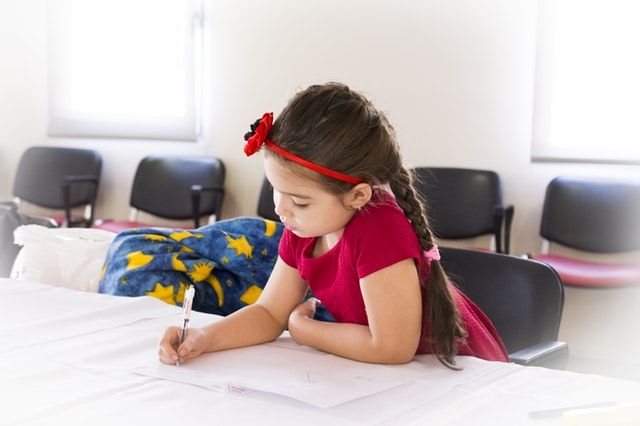
Following the success of introducing my children to computer programming with the Guessing Game project, I want to extend what we learned and make a spelling game. This post outlines the requirements for the game and looks at some of the libraries required.
Both my five-year-old son and seven-year-old daughter are showing signs of interest in what I do for a living: programming with computers. The world of finance, investments and insurance is unlikely to capture their attention, so I’ve been concentrating on showing them how to make simple games.
This game will work as a spelling test that can give them a chance to battle the computer and each other to get a high score. With luck, I can also engage them in the writing of the game and help them understand computer programming a little more.
The children are learning to spell and read but are at different levels. The game needs to be able to be set to the appropriate level so that they can both enjoy it.
What I want to do is create a simple game where the computer can ask them to spell a word, give a hint if necessary, and take their guess. We can gamify it a little by keeping track of scores and allowing them to level up.
We will add different types of hints: maybe to tell them the number of letters, to use the word in a sentence or to reveal a letter randomly.
Speech
A spelling test won’t work if the word appears and images are too prone to misinterpretation. What is required is for the computer to say the word out loud and for my children to type the response in using the keyboard.
My children are used to talking to the computer. We make a game of asking Siri questions on my iPhone to get help with things. So they are used to the way that the computer talks.
Siri is now available on my MacBook Air and this gives access to the voice synthesis. It is easy to get the computer to say “hello” at the command prompt with:
say hello
This can be called easily from Python using the subprocess
library separating the arguments from the program:
>>> from subprocess import call
>>> call(['say', 'This is being called from Python shell'])
It will also be possible to expand this game into different languages by making use of the pre-installed voices. These can be listed by typing say -v ?
. For example, to speak in French you can use Amelie’s voice:
say say -v Amelie 'Bonjour, je m''appelle Amelie'
This could be useful for doing spelling tests in different languages as they get older and take on subjects like French, German, Spanish or Italian.
Spellings
We could store spellings in a dictionary that contains the word and some examples of how to use the word in a sentence:
>>> words = {'cat': 'The cat meowed loudly and looked at its empty food bowl', 'dog': 'The dog did not like the postman and barked every time he delivered letters'}
The program can use a pseudo-random number generator to pick one of the spellings to ask. The randint
function is the same that we used in the Guessing Game project.
The first step is to get just the keys from the dictionary with list(words)
and then we can access individual words with an index which starts at zero and goes up to the length minus one. To randomly pick a work use randint(0, len(words)-1)
. Put together it looks like this:
>>> from random import randint
>>> word = list(words)[randint(0, len(words)-1)]
>>> word
'cat'
We can take input from the player and compare it back to the word – in the following example the word “cat” has been spelt incorrectly:
>>> guess = input("> ")
> kat
>>> guess == word
False
For the first iteration of the game, I will store the words in the file system in a filename containing the level of the words it contains. Level 1 will be monosyllabic words such as cat, dog, sit, etc.
Loading from file can be done with the json
package. This means that the files will look just like Python map and be easy to edit.
>>> import json
>>> f = open("dict.json", "r")
>>> words = json.load(f)
>>> f.close()
>>> words
{'cat': 'The cat meowed loudly and looked at its empty food bowl', 'dog': ['The dog did not like the postman and barked every time he delivered letters', 'The farmer used his dog to help bring in the sheep']}
Flow
The basic flow of the application will be as follows:
- Welcome the user and ask them to select a level between 1 and 10
- Load the dictionary for the level requested
- Pick a word from the dictionary
- Read out the word
- Ask the player to select an option: make a guess, get help, repeat the word, skip the word, or wants to quit the game
- Making a guess
- Read from the keyboard
- Compare with the word
- Give feedback
- Go back to step 4. in the outer loop if incorrect or forward to the scoring step if successful.
- Giving help options are as follows:
- To say how many letters are in the word
- Use the word in a sentence
- Reveal a letter, a new one each time this help is accessed
- Go back to step 4. in the outer loop
- A score should be awarded for the correct spelling, reduced if any of the letters were revealed.
- Repeating the word is straightforward and leads back to step 5.
- Skipping a word will give them zero points for the word and go back to step 3.
- Quitting will move to the following step
- When the game is quit, print out and say the overall score out of the total possible.
There are a few other things to consider. The same word should not be repeated within a test. If all the letters are revealed, the game should simply move on to the next word.
Coming Next
I’ll keep this post short, just covering the requirements. Over the next few posts I will document the complete project, first getting a basic working model up and running to keep the children interested by seeing the actual game. I’ll then break it down to be a little more modular and extend for different languages if there is time, or interest from the children.
My aim is to build in unit testing from the start using Python’s unittest
framework. This will be a first for me having never written test code for Python before. There is some learning I’ll have to do to get started.
I also want to help the children appreciate what test code does and how the test driven development approach works. They may not have the patience for that at this stage, so I’ll play it by ear.