Numbers and Strings in JavaScript
This is the first of a series of short posts about languages, patterns, frameworks or technologies that I have been exposed to recently for the first time.
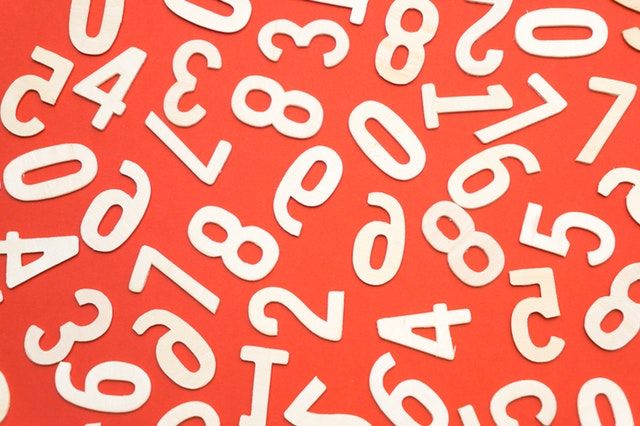
This is the first of a series of short posts about languages, patterns, frameworks or technologies that I have been exposed to recently for the first time.
Coming from a Java background, I have always been aware of JavaScript, but unwilling to dip into the language deeply. Although the names are similar, and they are both programming languages, the similarities stop there. There are a few things that Java developers need to know when first picking up JavaScript.
Going through the introductory training material will get you there but these courses tend to start from a zero-programming knowledge. I’ve extracted some interesting bits from my experience here.
Number or String
JavaScript is not strongly typed and exhibits a few strange behaviours compared to Java. The first is its ability to figure out the context of a number compared to a string. For example, in this snippet it can tell that the two variables should be treated as numbers, even though one has been initialised as a string:
var a = 4;
var b = "2";
var c = a / b;
c;
2
That is handy… but you have to be careful. The concatenation operator, just like in Java is the plus symbol, +
, and it will behave differently under similar circumstances to the first example. In the following case it will decide to concatenate rather than add the two numbers.
var a = 4;
var b = "2";
var c = a + b;
c;
"42"
Note that the other simple operators, minus and multiplies, behave like division so that real numbers and their string representations will work as expected.
The Same or Really the Same
So how do you compare a variable string that represents a number and its equivalent number? JavaScript introduces a new operator to handle the fact that sometimes the number should equal the string and sometimes it should not. Simple equality, as in Java, is done with the double equals, ==
. JavaScript will say the following two variables are the same.
var a = 42;
var b = "42";
var eq = a == b;
eq;
true
That might not be what we need, however, as we might want to check type also. Introducing the triple equals, ===
, operator. This will check both the type and value.
var a = 42;
var b = "42";
var eq = a === b;
eq;
false
One further point is that if you want to negate the above operator, there are also two choices for doing “not equals”. You can use an exclamation mark with equals (!=
) to negate the double equals, or the an exclamation mark with two equals (!==
) to negate the exactly equals. In both cases, the first equals symbol changed to !
.
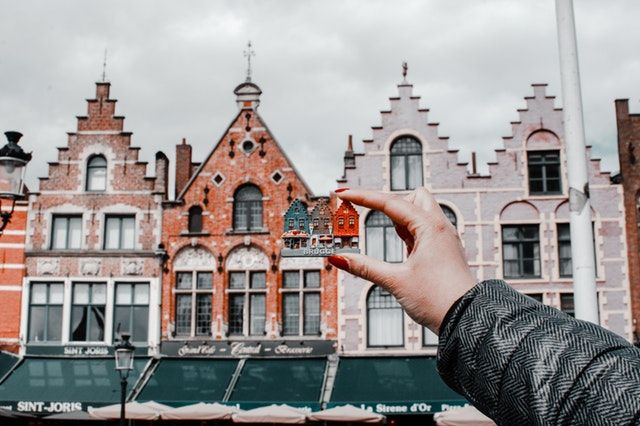